In a previous post “Customize the output of Powershell Gridview”, I showed how to create a Gridview script that would allow you to select files by type and delete them. In this post I will show how to add a popup window that will allow you to confirm your choice to delete. In that post I placed a series of files of different types in my C:\temp folder and then used Out-GridView with the -passthrough option to select certain files and delete them.
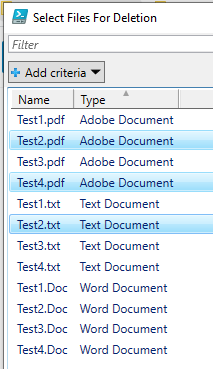
But what if you are like me and too quick to click buttons and accidentally do stuff you didn’t want to do? That’s where a confirmation popup comes in handy and I use them a lot in my code. Here is the code from the previous post:
$FILEPATH = “C:\TEMP”
Set-Location -Path $FILEPATH
$FILES= Get-childitem $FILEPATH |
SELECT Name, @{Name=”Type”;
Expression={
if($_.name -like ‘.doc’) {“Word Document”} if($_.name -like ‘.txt’) {“Text Document”}
if($_.name -like ‘*.pdf’) {“Adobe Document”}
}
} | Out-GridView -PassThru -Title “Select Files For Deletion”
Foreach ($SELECTION in $FILES)
{
Remove-Item $SELECTION.name
}
The basic rundown of this script is that it uses the Get-childitem commandlet to retrieve the list of files in the c:\temp folder, then uses the Expression command with an IF statement to create a column with file types. Then it uses the -passthrough option of Out-GridView to pass the filenames selected through to a Remove-Item command which deletes the files.
First we will add some code to the beginning of the script:
Add-Type -AssemblyName System.Windows.Forms
This will add the proper .NET Code class to the Powershell session.
Next will add some code between the Out-GridView code and the Remove-Item If statement. This will cause a window with yes/no buttons to popup before deleting the files. The button “answer” is passed to the variable $OUTPUT. If the value of $OUTPUT is “YES” then the code to delete the files (which will be placed inside this IF statement) will execute, otherwise the popup window will close and nothing will happen:
$OUTPUT=[System.Windows.Forms.MessageBox]::Show("Thomas, you are about to delete some important files, are you really, really sure you want to do this? Come on man!!!" , "WARNING!!!" , 4)
if ($OUTPUT -eq "YES" )
{
Foreach ($SELECTION in $FILES)
{
Remove-Item $SELECTION.name
}
}
Now when you execute the script, select some files for deletion and click ok, you will get this popup window:
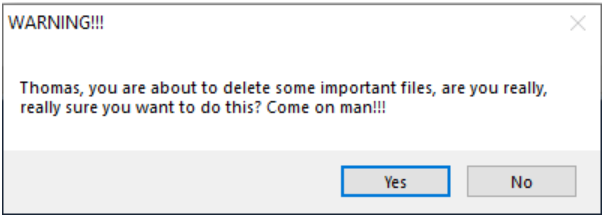
Here is the complete code:
Add-Type -AssemblyName System.Windows.Forms
$FILEPATH = “C:\TEMP”
Set-Location -Path $FILEPATH
$FILES= Get-childitem $FILEPATH |
SELECT Name, @{Name=”Type”;
Expression={
if($_.name -like ‘.doc’) {“Word Document”} if($_.name -like ‘.txt’) {“Text Document”}
if($_.name -like ‘*.pdf’) {“Adobe Document”}
}
} | Out-GridView -PassThru -Title “Select Files For Deletion”
$OUTPUT=[System.Windows.Forms.MessageBox]::Show("Thomas, you are about to delete some important files, are you really, really sure you want to do this? Come on man!!!" , "WARNING!!!" , 4)
if ($OUTPUT -eq "YES" )
{
Foreach ($SELECTION in $FILES)
{
Remove-Item $SELECTION.name
}
}